Mastering Object-Oriented Programming in Python: A Guide to Classes and Objects
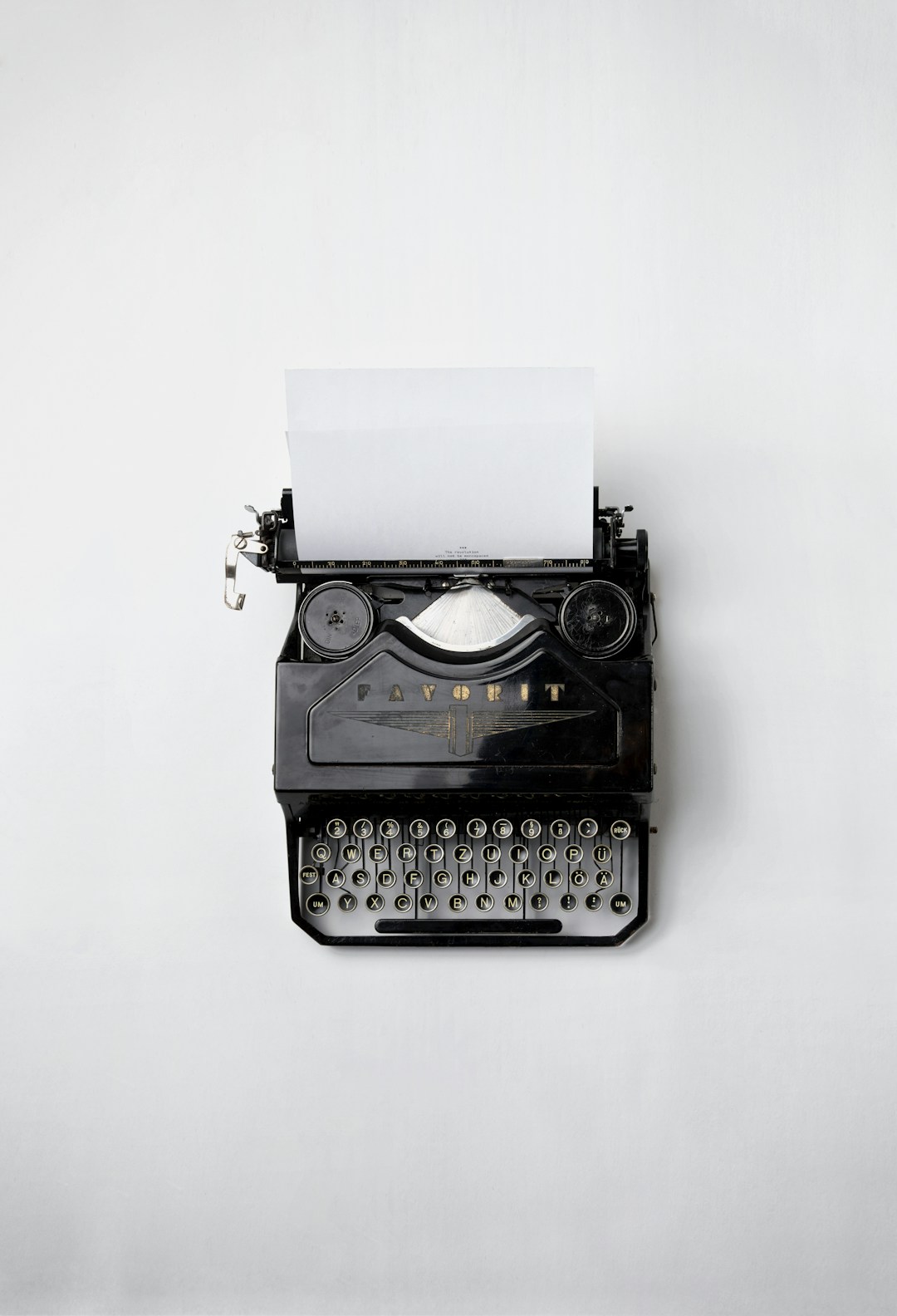
Introduction to Object-Oriented Programming
Object-Oriented Programming, commonly known as OOP, is a programming paradigm that uses objects and classes. It's one of the most popular and widely used styles of coding. Before diving in, I’ll admit—I once thought 'OOP' was a typo for 'oops!' I was wrong, by the way! So let's clear up any confusion and explore what OOP actually means and how it can benefit you as a programmer.
At its core, OOP is all about creating reusable code that is easy to maintain. Instead of writing the same code over and over again, you write a class once and then create multiple objects using that class. This makes your code more organized and modular, which is a fancy way of saying easier to manage.
### Key Concepts of Object-Oriented Programming
Let's break down some of the key concepts you need to know about OOP:
1. Classes and Objects: A class is like a blueprint for creating objects. Think of it as a recipe. An object, on the other hand, is an instance of a class—a cake made from that recipe.
2. Encapsulation: This means bundling the data and methods that work on the data within one unit, like a capsule. It helps protect the data from outside interference and misuse.
3. Inheritance: This allows new classes to borrow characteristics (fields and methods) of existing ones. Picture inheriting your grandma’s secret recipe book, and you can add your own twists without rewriting everything.
4. Polymorphism: This allows methods to do different things based on the object it is acting upon, even with the same name. Just like how a smartphone can be used to call, text, or play games—it adapts based on the situation.
### Example Code
To make things clearer, here's a simple example in Python:
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
raise NotImplementedError("Subclass must implement abstract method")
class Dog(Animal):
def speak(self):
return "Woof!"
class Cat(Animal):
def speak(self):
return "Meow!"
# Creating objects
dog = Dog("Buddy")
cat = Cat("Whiskers")
print(dog.speak()) # Outputs: Woof!
print(cat.speak()) # Outputs: Meow!
In this example, Animal
is the parent class with a method speak
that is meant to be overridden by its subclasses Dog
and Cat
. Now that's inheritance and polymorphism in action!
### Benefits of OOP
Object-Oriented Programming comes with a bunch of benefits, such as:
1. Reusability: Once a class is written, it can be used over and over again—just like a recipe!
2. Scalability: OOP makes it easier to manage larger projects. You can add new features without breaking existing ones.
3. Maintainability: Because of its organized structure, it’s easier to debug and find issues.
### Real World Application
OOP isn’t just a theoretical concept; it's used in real-world applications you likely use every day. Major software, games, and even mobile apps rely on OOP principles to function efficiently. From everything on your operating system to your favorite social media apps—they all use OOP!
Who knew that coding in OOP could be almost as fun as eating a homemade cake? Well, maybe not, but you get the point. Happy coding!
Defining Classes in Python
Alright, let's dive into the heart of object-oriented programming with Python: defining classes. Classes are essentially blueprints for creating objects. Python makes it delightfully simple, even for those of us who occasionally trip over our own feet in excitement!
To define a class in Python, we use the class
keyword. Here's a basic example:
class Dog:
def __init__(self, name, age):
self.name = name
self.age = age
def bark(self):
return "Woof!"
In this example, we've defined a simple Dog
class with two attributes: name
and age
. These attributes are initialized using a special method called __init__
. Essentially, __init__
is the constructor method that gets called when we create a new instance of the class.
Let's break it down:
- class Dog: Defines a new class called
Dog
. - def init(self, name, age): The constructor method.
self
refers to the instance of the class.name
andage
are parameters that initialize the object's attributes. - self.name = name: Assigns the value of the
name
parameter to thename
attribute of the instance. - def bark(self): A method that makes our dog bark. When called, it simply returns the string "Woof!".
Here's how you would actually use this in practice:
my_dog = Dog("Rex", 5)
print(my_dog.name) # Output: Rex
print(my_dog.bark()) # Output: Woof!
So, we've created an instance of the Dog
class named my_dog
. We passed the string "Rex" and the integer 5
to initialize the name
and age
attributes, respectively. By accessing my_dog.name
, we get the dog's name. Calling my_dog.bark()
makes our dog “bark”.
A Quick Note on self
If you're scratching your head over self
, don't worry—you're not alone. When I first started, I thought it was a little redundant (and frankly, it felt a bit like talking to myself). But it's a crucial part of how Python distinguishes between different instances of a class. Think of self
as a way for an object to refer to itself.
Adding More Functionality
You can add as many methods and attributes to a class as you need. Here, let's extend the Dog
class to include more details:
class Dog:
def __init__(self, name, age, breed):
self.name = name
self.age = age
self.breed = breed
def bark(self):
return "Woof!"
def get_info(self):
return f"{self.name} is a {self.age} year(s) old {self.breed}."
Not only can our dog bark, but now we can also get some additional information about it. We added a breed
attribute and a get_info
method.
my_dog = Dog("Rex", 5, "German Shepherd")
print(my_dog.bark()) # Output: Woof!
print(my_dog.get_info()) # Output: Rex is a 5 year(s) old German Shepherd.
Why Stick with Classes?
Classes in Python give you a structured way to manage and organize your code. They allow you to bundle data and functionality together, making your programs more modular and easier to understand.
So, if you've ever felt overwhelmed by big codebases, classes might just be your new best friend. And unlike a real-life dog, you don't have to take them for a walk!
Keep experimenting and creating new classes. The more you practice, the easier it will become. Python’s simplicity here is both a blessing and a curse—there’s no shortage of ways to take advantage of it, but sometimes that means there’s a lot to learn. Good luck, and happy coding!
Creating and Using Objects
Now that we've wrapped our heads around defining classes in Python, it's time to bring those classes to life by creating and using objects. As exciting as it may sound, this process is straightforward and immensely powerful. Trust me, after creating your first object, you'll wonder how you ever lived without it.
Creating an Object
To create an object, we need to call the class itself. Imagine the class as a blueprint and the object as a house built from that blueprint. For instance, if we have a class named Car
, creating an object would look something like this:
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
# Creating an object of the Car class
my_car = Car('Toyota', 'Corolla', 2019)
In the example above, my_car
is an object of the Car
class. The __init__
method runs when we create an instance, initializing the attributes make
, model
, and year
.
Accessing Object Properties
Once we have our object, we can access its properties using the dot notation. Think of it as reaching into a toolbox to get the specific tool you need. Here’s how it works:
print(my_car.make) # Output: Toyota
print(my_car.model) # Output: Corolla
print(my_car.year) # Output: 2019
Calling Object Methods
Just as you can access properties, you can also call methods on your object. Let’s assume our Car
class has a method that describes the car:
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
def describe(self):
return f'{self.year} {self.make} {self.model}'
# Creating the object again
my_car = Car('Toyota', 'Corolla', 2019)
# Calling the describe method
print(my_car.describe()) # Output: 2019 Toyota Corolla
Modifying Object Properties
Objects are flexible, just like my diet on weekends. You can modify their properties at any time after creation:
my_car.year = 2020
print(my_car.year) # Output: 2020
Deleting Object Properties
Sometimes, you may need to delete an attribute from an object. This can be done using the del
keyword:
del my_car.model
# Trying to access it now will raise an AttributeError
# print(my_car.model) # Uncommenting this will raise an error
Object Comparison and Identity
It’s often useful to compare objects. Two objects are the same if they refer to the same instance in memory. Use the is
keyword for this.
another_car = my_car
print(my_car is another_car) # Output: True
# Create a new object with the same attributes
yet_another_car = Car('Toyota', 'Corolla', 2019)
print(my_car is yet_another_car) # Output: False
The __str__
and __repr__
Methods
These special methods allow us to define how objects are printed. While __str__
is meant for end-user readability, __repr__
is intended for developers. Let’s add these to our Car
class:
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
def describe(self):
return f'{self.year} {self.make} {self.model}'
def __str__(self):
return f'[{self.year}] {self.make} {self.model}'
def __repr__(self):
return f'Car({self.make!r}, {self.model!r}, {self.year!r})'
my_car = Car('Toyota', 'Corolla', 2019)
print(str(my_car)) # Output: [2019] Toyota Corolla
print(repr(my_car)) # Output: Car('Toyota', 'Corolla', 2019)
Objects make your code neater and your life easier. Embrace them like a long-lost sibling!
Advanced Concepts: Inheritance and Polymorphism
After getting comfortable with the basics of object-oriented programming (OOP) in Python, it's time to dive into some of the more advanced concepts like inheritance and polymorphism. These might sound a bit intimidating at first, but trust me, they will make your code more efficient and easier to manage. Let's take this step by step, shall we?
What is Inheritance?
Inheritance is one of the core concepts of OOP. It allows one class (the child or subclass) to inherit the attributes and methods of another class (the parent or superclass). This helps in promoting code reusability. Think of it like inheriting traits from your parents - only in code form.
In Python, you can achieve inheritance like this:
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
pass
class Dog(Animal):
def speak(self):
return "Woof!"
class Cat(Animal):
def speak(self):
return "Meow!"
# Creating objects
dog = Dog("Buddy")
cat = Cat("Whiskers")
print(dog.speak()) # Output: Woof!
print(cat.speak()) # Output: Meow!
In the example above, the Dog
and Cat
classes inherit from the Animal
class. Both override the speak
method. This is a simple way to manage and organize code better.
Why Use Inheritance?
Here are some reasons why you might want to use inheritance: - Code Reusability: You don't have to write the same code again. - Ease of Maintenance: Changes in the parent class can propagate to all derived classes. - Logical Representation: Better representation of real-world relationships.
What is Polymorphism?
Polymorphism is another fundamental concept in OOP that allows objects of different classes to be treated as objects of a common superclass. It's like when you know several languages; you can communicate in different ways but still understand the context.
In Python, polymorphism is usually achieved by method overriding. Let's revisit our animal example:
def make_animal_speak(animal):
print(animal.speak())
make_animal_speak(dog) # Output: Woof!
make_animal_speak(cat) # Output: Meow!
In this example, the make_animal_speak
function can take any object that has a speak
method, thanks to polymorphism. Neat, right?
Method Overriding vs Overloading
- Method Overriding: Happens in inheritance where the subclass implements a method already provided by one of its parent classes. This allows a subclass to provide a specific implementation of a method that is already defined.
- Method Overloading: Although not fully supported in Python, method overloading allows a class to have multiple methods with the same name but different arguments.
Unfortunately, Python doesn't support method overloading directly, but you can still use default arguments to achieve a similar effect:
class Example:
def add(self, a, b, c=0):
return a + b + c
example = Example()
print(example.add(1, 2)) # Output: 3
print(example.add(1, 2, 3)) # Output: 6
Using the super()
Function
While discussing inheritance, I can't forget to mention the super()
function. This built-in function allows you to call methods from a parent class. It's particularly useful when you want to extend the functionality of the inherited methods.
class Parent:
def __init__(self):
print("Parent class initialized")
class Child(Parent):
def __init__(self):
super().__init__()
print("Child class initialized")
child = Child()
# Output:
# Parent class initialized
# Child class initialized
The super().__init__()
call initializes the parent class before anything else happens in the child class. It's an essential tool for working with inheritance in a concise and pythonic way.
Practical Applications
Knowing when and how to use inheritance and polymorphism can be a game-changer in your coding journey. They are extensively used in frameworks and libraries, such as Django, Flask, and even in machine learning libraries like TensorFlow and PyTorch.
Here’s a quick table to highlight the differences:
Concept | Definition | Example Code |
---|---|---|
Inheritance | Child class inherits from parent class | class Dog(Animal): |
Polymorphism | Different classes have a common interface | def make_animal_speak(animal): |
Method Overriding | Subclass offers specific implementation | def speak(self): return "Woof!" |
I hope this breakdown helps you understand these advanced concepts more clearly. Remember, the more you practice, the more naturally these ideas will come to you.
And hey, don't worry if it takes a while to get the hang of things. Even Einstein had to start somewhere!
Practical Applications of OOP in Python
In the previous chapters, we learned about the foundational concepts of object-oriented programming (OOP) in Python. Now, let's delve into the practical applications of OOP. This is where the fun really begins! You’ll see how OOP can be useful in real-world scenarios by making your code more modular, maintainable, and scalable. I'll walk you through some examples so you can see the practicality of these concepts in action.
1. Web Development
Let's start with web development, one of the most common areas where OOP shines. Frameworks like Django and Flask are built on OOP principles. By using classes and objects, these frameworks help you manage different components of a web application like databases, user interfaces, and server requests. For instance, you can define a User
class to handle all user-related functionalities, such as registration, authentication, and profile management.
python<br>class User:<br> def __init__(self, username, email):<br> self.username = username<br> self.email = email<br><br> def register(self):<br> # Code to register the user<br> pass<br><br> def login(self):<br> # Code to authenticate the user<br> pass<br><br> def update_profile(self, new_email):<br> self.email = new_email<br> # Code to update the user's profile<br> pass<br>
The above example demonstrates how you can encapsulate user functionalities into a single class, making your code modular and easy to manage.
2. Game Development
OOP is indispensable in game development. Libraries like Pygame make extensive use of OOP to manage game elements such as characters, enemies, and environments. In a game, you might have a Character
class with methods for movement, attack, and defense.
python<br>class Character:<br> def __init__(self, name, health):<br> self.name = name<br> self.health = health<br><br> def move(self, direction):<br> # Code to move the character<br> pass<br><br> def attack(self, target):<br> # Code to attack an enemy<br> pass<br><br> def defend(self):<br> # Code to defend against an attack<br> pass<br>
With OOP, you can easily reuse and extend your code. For example, you can create a Hero
class that inherits from Character
and adds special abilities.
3. Data Science
Data science often involves working with complex datasets and algorithms, making OOP a valuable tool. Classes can be used to represent datasets and encapsulate data manipulation methods. For example, you can create a Dataset
class to load and process data.
python<br>class Dataset:<br> def __init__(self, data):<br> self.data = data<br><br> def preprocess(self):<br> # Code to preprocess the data<br> pass<br><br> def analyze(self):<br> # Code to perform data analysis<br> pass<br><br> def visualize(self):<br> # Code to visualize the data<br> pass<br>
This approach makes your code more organized and easier to understand, especially when working on large projects.
4. Desktop Applications
If you’ve ever built a desktop application, you know how quickly it can become complex. OOP can simplify your life here too. Using libraries like Tkinter, you can create reusable components for your GUI elements. For instance, you might create a Button
class to handle button-specific properties and methods.
python<br>import tkinter as tk<br><br>class Button(tk.Button):<br> def __init__(self, master, text, command):<br> super().__init__(master, text=text, command=command)<br><br> def set_color(self, color):<br> self.config(bg=color)<br> # Additional code to handle button color<br> pass<br>
By encapsulating button-specific logic within a class, you can easily create and manage multiple buttons in your application.
5. Automation Scripts
Last but not least, OOP can be a lifesaver when writing automation scripts. Imagine you need to scrape multiple websites. Instead of writing repetitive code, you can create a Scraper
class and reuse it for different websites.
python<br>import requests<br><br>class Scraper:<br> def __init__(self, url):<br> self.url = url<br><br> def fetch_content(self):<br> response = requests.get(self.url)<br> return response.text<br><br> def parse_content(self, content):<br> # Code to parse the content<br> pass<br><br> def save_data(self, data):<br> # Code to save the data<br> pass<br>
This not only saves you time but also makes your code more flexible and easier to update.
As you can see, OOP in Python is not just a theoretical concept. It has numerous practical applications that can make your programming journey more efficient and enjoyable. If you haven’t already, give these examples a try and see how OOP can transform your approach to coding.
Conclusion and Best Practices
Object-Oriented Programming (OOP) is not just a programming style; it's a paradigm that helps us think about software design in a more organized and modular way. Throughout this blog series, we've delved into the fundamentals and advanced concepts of OOP, specifically in Python. As we wrap things up, let's recap some best practices and highlight crucial insights to truly master OOP in Python.
Consistency is Key
When writing classes and objects, maintaining a consistent style is crucial. Consistent naming conventions, indentation, and documentation make your code more readable and more easily maintained. As they say, code should be written for humans to read and only incidentally for machines to execute.
Leverage Built-in Functions
Python provides numerous built-in functions and libraries that can simplify your OOP code. Methods such as __str__
and __repr__
for string representation of objects, and __eq__
for object comparison, can make your classes more intuitive and user-friendly.
Use Inheritance Wisely
While inheritance is a powerful feature in OOP, it can easily lead to complexities if not used properly. Aim to keep your inheritance hierarchies shallow and favor composition over inheritance. Overusing inheritance can lead to tightly coupled code, making maintenance and debugging a nightmare. Yes, I learned this the hard way.
Encapsulate Data
One of the pillars of OOP is encapsulation, which involves bundling the data with the methods that operate on that data. This means you should keep your data members private and use public methods to access and modify them. Encapsulation ensures that your objects maintain a valid state and adhere to the principle of least privilege.
Polymorphism is Your Friend
Polymorphism allows for methods to operate on objects of different classes, as long as they follow a particular interface. This can significantly reduce code redundancy. For example, if you have a method draw_shape
that can draw circles, squares, and triangles, you only need to write that method once if your shapes adhere to a common interface.
Principle | Description | Benefit |
---|---|---|
DRY (Don't Repeat Yourself) | Avoid code duplication by using methods, classes, and modules. | Reduces errors and improves code maintainability. |
YAGNI (You Ain't Gonna Need It) | Don't add functionality until it is necessary. | Keeps the codebase clean and manageable, without unnecessary complexity. |
KISS (Keep It Simple, Stupid) | Strive for simplicity in your code. Complex solutions are not always better. | Simplifies understanding and maintenance. |
SOLID Principles | Follow the five SOLID principles of OOP (Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, Dependency Inversion). | Encourages a robust, scalable, and maintainable codebase. |
Document Your Code
Clear documentation is essential for maintaining and scaling your projects. Use docstrings to explain what a class or a method does, its parameters, and return values. This practice not only helps others understand your code but also acts as a guide for yourself when you revisit your code after a long time.
Write Unit Tests
Always write unit tests for your classes. Testing ensures that your code behaves as expected and helps catch bugs early. Python's unittest
library or third-party tools like pytest
are great starting points. And trust me, having tests can save you from pulling your hair out when a bug appears out of nowhere.
In conclusion, mastering OOP in Python takes practice and dedication. Following these best practices will help you write cleaner, more efficient, and sustainable code. So, the next time you sit down to write Python code, remember these tips, and you'll find yourself writing better, more robust software.
Object-Oriented Programming
Python
Classes
Objects
Coding
Programming